JavaScript Interview Questions and Answers 2023
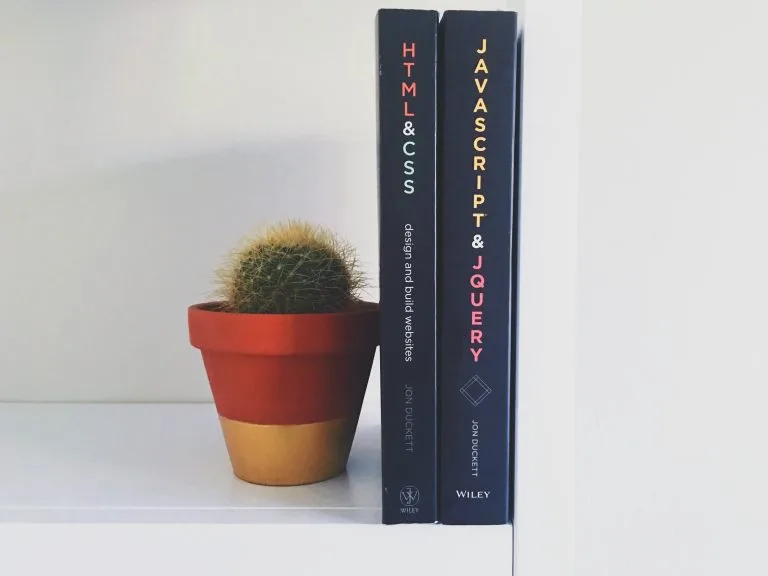
Companies such as Facebook and Google use JavaScript to develop complex web applications with ease. With time, the popularity of the language will only increase and so will the demand for JavaScript developers. Keeping this as one of the reasons, we designed this article outlining the top JavaScript Interview Questions for you to consider when preparing for the interview.
JavaScript Interview Questions: Beginners Level
- What is the difference between null and undefined?
- What are the different data types available in JavaScript?
- Explain Closures in JavaScript
- Difference between “==” and “===” operators.
- Explain call(), apply() and, bind() methods.
- What is DOM? How to create a DOM element?
- What is the difference between keywords let, const, and var?
- How is for..in loops different from for..of loops?
- What are the different ways to remove array elements in JavaScript?
- Explain the use of an instanceof operator in JavaScript.
- What is Scope?
- What is the difference between JavaScript and AJAX?
- What are the differences between while and do-while loops in JavaScript?
- What is the disadvantage of creating a true private method?
JavaScript Interview Questions: Advanced Level
- What is the difference between the private variable, public variable, and static variable?
- Explain the difference between synchronous and asynchronous functions.
- How to use Fetch with async-await?
- Explain the concept of hoisting in JavaScript?
- In AJAX, what are the ready states of a request?
- Explain types of postback in AJAX?
JavaScript Interview Questions: Beginners Level
1. What is the difference between null and undefined?
A null is an object and ideally means nothing. On the other hand, undefined is a variable that has no assigned value. That is to say, we explicitly assign null to a variable whereas undefined is a plain and simple declaration of a variable.
Example for null and undefined in JavaScript:
var x; // x is a variable var y; // y is a variable x = null; // x is null // If we test the type of both the variables, console.log(x); //Output: object console.log(y); // Output: undefined
2. What are the different data types available inJavaScript?
Number: Number data type supports both integers and floating-point values. In addition to this, JavaScript also houses special numbers such as NaN, Infinity, etc.
String: A sequence of characters surrounded by quotes (single or double or backticks). Where single and double quotes are practically the same, backticks help to add variables or expressions within a string.
// Example:
let name = "JavaScript Interview Questions";
// embedding a variable
alert( Learn ${name}!
); // Learn JavaScript Interview Questions!
Boolean: The logical data type with two possible values: true and false.
// Example: let value = 4 > 1; alert( value ); // true
Undefined: Undefined is a special data type for variables  that are declared but not defined.
Null: Null is the datatype for unknown values.
Symbol: Unique identifiers fall under the category of a symbol.
Object: These are complex and used for storing values of more than one type.
3. Explain Closures in JavaScript
JavaScript closure is a unique feature where variables outside a current scope can be easily accessed by functions in the inner scope. Technically, closures are created after every function definition in JavaScript. The best way to understand the concept of closure function in JavaScript is to define a function within a function and use it to display variable values of the outer function.
// Example: function testJ() { var title = 'JavaScript Closure'; var welcome_msg = 'Hello! This is a list of top JavaScript Interview Questions'; function displayTitle() { alert(welcome_msg); alert(title); } return displayTitle; } var myTitle = test J(); myTitle();
The scope of a variable is within the function definition. However, in JavaScript, closures extend the scope, allowing the function displayTitle to retain the reference to the title variable. When the interpreter invokes myTitle, the title variable is still in reference,  then passed to alert, which ultimately gives the final output.
4. Difference between “==” and “===” operators.
== operator checks the value of two variables disregarding the type. However, === takes into consideration the value as well as the type of the variable.
If we compare:
"7" == 7 // returns true
"7" === 7 // returns false.
5. Explain call(), apply() and, bind() methods.
The 'this' keyword refers to the immediate owner of a function. Now the call(), apply(), and bind() methods help decide how to set the '  this' keyword as and when the interpreter calls a function.
- Bind( ): This method will first create a new function and then sets the keyword to a specific object.
Syntax: function.bind(thisArg, optionalArguments)
- Call(): Unlike the Bind() method, the call() method doesn't create a copy of the function. Instead, it sets this keyword within the existing function and executes the same instantly.
Syntax: function.call(thisArg, arg1, agr2, …)
- Apply(): This is partially the same as that of the call() method. The two differ in the sense that apply() accepts an array as an argument whereas call() accepts one or more arguments separated by a comma.
Syntax: function.apply(thisArg, [argumentsArr])
Find a detailed guide on the three methods here.
6. What is DOM? How to create a DOM element?
DOM is the acronym for the Document Object Model. It is the application programming interface for both the HTML and the XML documents. Using DOM, we can define the document structure and ways in which the same can be modified or manipulated.
Primarily, XML is used to represent information stored within varied systems. Most of these are in the form of documents. DOM here helps manage the documents and access the data present within. With DOM, developers can easily create documents, specify their structure, and also add/modify/delete elements.
Example:
<div id="div1"> <p id="p1">This is the first paragraph.</p> <p id="p2">This is the second paragraph.</p> </div> <script> var test = document.createElement("t"); var node = document.createTextNode("Creating DOM element in JavaScript."); test.appendChild(node); var element = document.getElementById("div1"); element.appendChild(test); </script>
The above example creates a DOM element t. To add value to the element, we first create a node and then append the same using the appendChild method.
The second half of the example traces an existing element and then appends the test element to the new one.
7. What is the difference between keywords let, const, and var?
The keyword var helps declare variables and even initialize them. By default, the scope of variables declared using the var keyword is either functional or global.
Example:
function test() { var x = 10; console.log(x); // output 10 if (true) { var x = 20; console.log(x); // output 20 } console.log(x); // output 20 }
The keyword 'let' is similar to 'var' in the sense that it allows you to define a variable and also initialize it. The difference lies in the scope of the variable. Unlike var, the scope of variables defined using the let keyword remains within the block it is created.
Example:
function test() { let x = 10; console.log(x); // output 10 if (true) { let x = 20; console.log(x); // output 20 } console.log(x); // output 10 }
The keyword const facilitates the creation of variables with fixed values. Meaning that once a value is assigned to the const variable, it cannot be reassigned.
Example:
function test() { const X = 10; //Conventionally variables defined using const keyword to be in UPPERCASE console.log(X); // output 10 const X = 20; // Throws TypeError }
8. How is for..in loops different from for..of loops?
Both for..in and for..of loops iterate over an array or the object. The difference lies in what they iterate. The for..in loops scans through the object iterating over the enumerable properties. The loop focuses on the property only.
On the other hand, the for..of loops emphasizes the values in the defined objects.
Example: for..in loops
<html> <body> <script> Object.prototype.inherProp1 = function() {}; Array.prototype.inherProp2= function() {}; var lang = ["Java", "Python", "JavaScript"] lang.fourthLang = "C"; for (var key in lang) { if (org.hasOwnProperty(key)) { document.write(key); document.write("</br>"); } } </script> </body> </html> Output: 0 1 2 fourthLang
Example: for..of loops
<html> <body> <script> var lang = ["Java", "Python", "JavaScript"] for (var key of lang) { document.write(key); document.write("</br>"); } </script> </body> </html> Output: Java Python JavaScript
9. What are the different ways to remove array elements in JavaScript?
To remove elements from JavaScript array, follow any of the said methods:
Pop: To remove the last element.
var my_arr = [1, 2, 3, 4]; my_arr.pop(); // returns 4 console.log( my_arr ); // [1, 2, 3]
Shift: To remove elements from the beginning of the array. The shift method is the same as that of pop with the only difference being the position of removal.
var my_arr = [1, 2, 3, 4]; my_arr.shift(); // returns 1 console.log( my_arr ); // [2, 3, 4]
Splice: To remove elements from an array irrespective of the position. In other words, the splice method removes elements from anywhere within the array. It is important to specify the position or the index location of the element for removing them from the array. The splice method takes three arguments. The first one specifies the start position, the second tells how many elements are to be removed, and the third (optional) indicates the elements that will be added to the JavaScript array.
var my_arr = [1, 2, 3, 'JavaScript Interview Questions', 'Python For Machine Learning']; console.log(my_arr.splice(0,3)); // [1,2,3] console.log( my_arr ); //['JavaScript Interview Questions', 'Python For Machine Learning']
Other methods used to remove elements from a JavaScript array:
Length: Simply edit the length of the array to remove the last few elements.
var my_arr = [1, 2, 3, 4, 5, 6, 7, 8]; my_arr.length = 4; // removes elements console.log( my_arr ); // [1, 2, 3, 4]
Delete: Specify the index to remove the element. Note that the delete function will not edit or modify the length of the array.
var my_arr = [1, 2, 3, 4]; delete my_arr[1]; console.log( my_arr ); // [1, undefined, 3, 4]
10. Explain the use of an instanceof operator in JavaScript.
At times, we need to check the type of an object to verify whether it belongs to a particular function or not. The instanceof operator in JavaScript helps with the same. Technically, the instanceof operator returns a Boolean value depending upon whether the constructor prototype is of type object.
Example 1:
function Car(type, mod_no, color) { this.type = type; this.mod_no = mod_no; this.color = color; } const my_car = new Car('Honda', 'X8123', 'Grey'); console.log(my_car instanceof Car); // expected output: true console.log(my_car instanceof Object); // expected output: true console.log(my_second_car instanceof Car); // expected output: false
Example 2:
// define constructors function f1() {} function f2() {} let obj1 = new f1() obj1 instanceof f1 // true, because: Object.getPrototypeOf(obj1) === f1.prototype // false, because f2.prototype is nowhere in obj1's prototype chain obj1 instanceof f2 obj1 instanceof Object // true
11. What is Scope?
In simple terms, scope determines the accessibility of variables within a JavaScript code. Conventionally, variables or functions in JavaScript have three major scopes.
Block Scope: Variables are accessible within the block they are defined. When we define a variable using either of the const and let keyword, they have block scope.
Global Scope: Variables with global scope can be accessed anywhere within the code. They are traditionally declared and defined in the global namespace.
Functional Scope: When we define a variable inside a function, it has a scope confined to the same. If we try to access the variable outside the function, the interpreter returns an error.
12. What is the difference between JavaScript and AJAX?
JavaScript is a client-side scripting language. The main purpose of the language is to create and complete tasks when designing a web page. Ajax, a part of JavaScript programming, is a technology that helps display asynchronous data without loading and reloading the entire page.
13. What are the differences between while and do-while loops in JavaScript?
JavaScript loops are the same as others. Concerning the while loop, we test the condition first and then execute the statements. However, with respect to do-while loops, the interpreter will first execute the statements and then test the condition. Meaning that at least for once the statement executes, even though the condition fails to satisfy.
14. What is the disadvantage of creating a true private method?
Creating a true private method results in the creation of a copy of the method. Now, these duplicate methods are not collected by the garbage collector, leaving them within the memory. Only when the object is destroyed will these empty the memory spaces.
JavaScript Interview Questions: Advanced Level
15. What is the difference between the private variable, public variable, and static variable?
Private, public, and static variables are related to OOPS and determine how and where the variables are used.
Private Variable
This is defined using the var keyword and the main reason here is to limit the scope. In simple terms, private variables are inaccessible by the objects of the class.
Public Variables
As the name says, public variables are open for use. Any object or function outside the class definition can access these variables and operate on them.
Static Variables
These are specific variables that answer only to the class. It is the class that owns the static variables. If at any point in time, you need to access the static variables, you must do it through the class name.
16. Explain the difference between synchronous and asynchronous functions.
Synchronous and asynchronous functions are two different concepts through which the interpreter executes statements with a function. To put it this way, when the interpreter executes in a sequential manner (one line after the other), it is synchronous. However, when the line of the code redirects the interpreter to a statement outside the function, it is asynchronous programming.
While JavaScript synchronous functions seem relevant, asynchronous functions keep the program running, even if a few lines of code are pending for execution.
17. How to use Fetch with async-await?
Async and await are the recent additions made in the JavaScript language back in 2017. Asynchronous functions have an additional keyword async right before the function name. The core idea here is that the function returns a promise.
For example:
async function my_func()
{
return 0;
}
my_func().then(alert); // 0
The function above returns a promise along with the output of 0.
Similar to the above, await is a keyword in JavaScript that forces the program to wait till the time the promise resolves. Only after the promise settles and the desired result is passed will the program continue its execution.
Example:
async function my_func() { let my_promise = new Promise((resolve, reject) => { setTimeout(() => resolve("done!"), 1000) }); let result = await my_promise; // wait until the promise resolves (*) alert(result); // "done!" } my_func();
The function above will pause at (*). After the promise fulfills, it continues with other lines of code. In other words, the JavaScript await keyword temporarily suspends the functioning of a program.
Fetch is a unique concept in JavaScript that helps in sending requests to the server and getting information from the same. Whether you want to load user information or submit an order or get updates from the server, the JavaScript fetch method facilitates all.
Example:
let my_response = await fetch(url); if (my_response.ok) { // if HTTP-status is 200-299 // get the response body (the method explained below) let json = await my_response.json(); } else { alert("HTTP-Error: " + my_response.status); }
18. Explain the concept of hoisting in JavaScript?
Hoisting is a unique feature that moves all of the variables declared in the piece of code to the top. This means that if you use variables even before their declaration, JavaScript doesn't result in an error.
For example:
a = 7; // Assign 7 to a elem = document.getElementById("demo"); // Find an element elem.innerHTML = a; // Display a in the element var a; // Declare a
The code above uses the variable a even before its declaration and runs without any error. Hoisting specifically arranges the code to move up all the declarations and then execute one after the other.
Note: When you declare variables using the let and const keyword, it is important to declare the keyword before using it in the program.
my_Car = "Honda";
alert(carname);
let my_Car;
Running the code above would return an error.
19. In AJAX, what are the ready states of a request?
There are 5 ready states of a request. By definition, readyState shows the state of a request.
- 0 for UNOPENED: open() is not called.
- 1 for OPENED: open() called but send() not called.
- 2 for HEADERS_RECEIVED: calling send() with information on status and headers.
- 3 for LOADING: To download data with the same held in responseText.
- 4 for DONE: Operation complete.
20. Explain types of postback in AJAX?
Ajax has two different postback options: Synchronous and Asynchronous.
Synchronous postback blocks the client till the time an operation completes. Whereas, asynchronous postback doesn't block the client.
We hope that these JavaScript Interview Questions will help you prepare for the interview. One thing to note is that the above isn't a finite list and there can be more. In case you have attended any JavaScript Interview in the past, and came across a different question, do let us know in the comment box.
Backend Technology Interview Questions
C Programming Language Interview Questions | PHP Interview Questions | .NET Core Interview Questions | NumPy Interview Questions | API Interview Questions | FastAPI Python Web Framework | Java Exception Handling Interview Questions | OOPs Interview Questions and Answers | Java Collections Interview Questions | System Design Interview Questions | Data Structure Concepts | Node.js Interview Questions | Django Interview Questions | React Interview Questions | Microservices Interview Questions | Key Backend Development Skills | Data Science Interview Questions | Python Interview Questions | Java Spring Framework Interview Questions | Spring Boot Interview Questions.
Frontend Technology Interview Questions
HTML Interview Questions | Angular Interview Questions | CSS Interview Questions
Database Interview Questions
SQL Interview Questions | PostgreSQL Interview Questions | MongoDB Interview Questions | MySQL Interview Questions | DBMS Interview Questions
Cloud Interview Questions
AWS Lambda Interview Questions | Azure Interview Questions | Cloud Computing Interview Questions | AWS Interview Questions
Quality Assurance Interview Questions
Moving from Manual Testing to Automated Testing | Selenium Interview Questions | Automation Testing Interview Questions
DevOps and Cyber Security Interview Questions
DevOps Interview Questions | How to Prevent Cyber Security Attacks | Guide to Ethical Hacking | Network Security Interview Questions
Design Product Interview Questions
Product Manager Interview Questions | UX Designer Interview Questions
Interview Preparation Tips
Strength and Weakness Interview Questions | I Accepted a Job Offer But Got Another Interview | Preparation Tips For the Virtual Technical Interview | 7 Tips to Improve Your GitHub Profile to Land a Job | Software Engineer Career Opportunities in Singapore | What can you expect during a whiteboard interview | How To Write A Resignation Letter | Recommendation Letter Templates and Tips.
Quick Links
Practice Skills | Best Tech Recruitment Agency in Singapore, India | Graduate Hiring | HackerTrail Litmus | Scout - Sourcing Top Tech Talent in ONE Minute | About HackerTrail | Careers | Job Openings.