Angular Interview Questions and Answers 2023
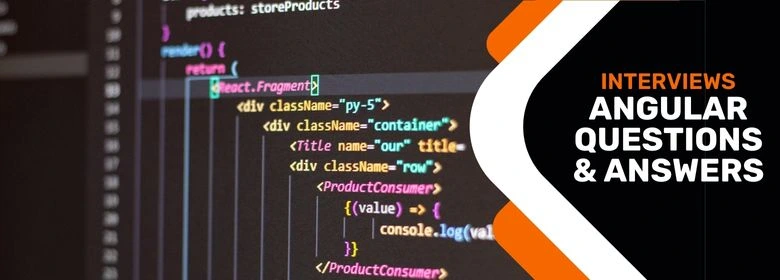
Today, Angular has become an in-demand web development platform for building efficient single-page applications. As a web developer, whether you want to pick up Angular or brush up on some Angular topics for an interview, you have come to the right place. In this article, we have compiled the answers to the commonly asked Angular interview questions. This will also help you understand which parts of the Angular framework you need to focus on.
Basic Angular Interview Questions
- What is Angular? How is it different from AngularJS?
- What are lifecycle hooks in Angular? Explain the lifecycle hooks in the order of execution.
- What are the building blocks of Angular?
- Explain the following terms in Angular: expressions, scope and text interpolation.
- What are some advantages and disadvantages of Angular?
- What are the differences between Angular decorator and annotation?
- What is data binding and what are its types?
Intermediate Angular Interview Questions
- Is it possible for an Angular application to render on the server-side?
- How can you communicate between application modules using core Angular functionalities?
- What is ahead-of-time(AOT) compilation in Angular? What are its advantages?
- What is MVVM in Angular?
- What is Angular Bootstrap?
- What is dirty-checking in Angular?
- Explain the process of digest cycle in Angular
Advanced Angular Interview Questions
- Explaining-app directive in Angular.
- How do Observable and Promise differ in execution?
- How do you get, set and clear cookies in Angular?
- How to hide a HTML element through a simple button click in Angular?
- What is an Angular Material Table?
Basic Angular Interview Questions and Answers
1. What is Angular? How is it different from AngularJS?
Angular is an open-source component-based framework for front-end web application development. It is built on TypeScript and is developed and maintained by Google. By using Angular, web developers and front-end developers can curate scalable cross-application platforms easily and effortlessly. It includes a wide range of libraries with features like client-server communication, routing, form management, etc. It also offers powerful features like dependency injection, declarative templates, and end-to-end tooling to develop, build, test, execute, and update the code.
Apart from knowing about what is Angular, you also need to know the difference between Angular and AngularJS. They are both open-source frameworks for front-end web application development, but have some major differences as listed below:
Parameters | AngularJS | Angular |
Architecture | Follows Model-View-Control design pattern | Uses components and directives |
Language | Built on Javascript | Built on Typescript, a superset of Javascript |
Mobile Support | No | Yes |
Structure | Difficult to maintain large applications | Structure designed to handle large applications |
Dependency Injection(DI) | Does not support DI | Supports hierarchical DI |
Support | No support available for AngularJS | Active support community and frequent updates |
Version | AngularJS refers to all the Angular versions v1.x | From version 2 onwards, the JS was dropped from AngularJS and it became Angular. |
2. What are lifecycle hooks in Angular? Explain the lifecycle hooks in the order of execution.
The lifecycle of a component instance starts when Angular instantiates the component class and creates its parent and child views. The component instance goes through various life cycle phases and at each phase you can control your application through certain timed methods known as hooks. Angular continuously checks for changes in the data-bound properties and executes the hooks according to this change detection. For each lifecycle event, you can implement one or more hook interfaces in the core Angular library.
After your application instantiates a component or directive by calling its constructor, Angular calls the hook methods you have implemented at the appropriate point in the lifecycle of that instance.
After instantiating a component or directive, Angular calls and executes the lifecycle hook methods in the following sequence:
Hook Method | Purpose | Called |
ngOnChanges() | The method executes whenever any data-bound input property is set or reset. It receives a ‘SimpleChanges’ object containing current and previous property values
Every operation performed here impacts performance significantly as the data changes happen too frequently |
Before ngOnInit() or whenever there is a change detection in properties
If your component has no input then ngOnChanges() will never execute |
ngOnInit() | To initialise the component or directive | Only once after the ngOnChanges() |
ngDoCheck() | When Angular can’t detect the changes, this hook will detect and act on the identified changes | Right after ngOnChanges() after each change detection run and immediately after first run of ngOnInit() |
ngAfterContentInit() | Executes when external content is projected into the component or directive view | Only once after the initial ngDoCheck() |
ngAfterContentChecked() | Executes after the projected content is checked | After ngAfterContentInit() and subsequent ngDoCheck() |
ngAfterViewInit() | Executes after the component views, child views and directive are initialised | Only once after the first ngAfterContentChecked() |
ngAfterViewChecked() | Executes after the component views, child views and directive are checked | After the ngAfterViewInit() and after subsequent ngAfterContentChecked() |
ngOnDestroy() | This is the last cleanup hook which is executed right before Angular destroys the component, child views and directive. | Right before destroying the component or directive |
3. What are the building blocks of Angular?
Following image shows the core building blocks in Angular and how they are related:
Let’s now understand each of these blocks:
- Components: Components compose an application and can control several views. An Angular application must have at least one root component consisting of a TypeScript class with a @Component() decorator, an HTML template, and styles. This model provides powerful encapsulation and a user-friendly application structure. Components also make it easy to unit test your application and increase the readability of your code. The application logic is written in the main application class and this class communicates with the views through API’s methods and properties.
- Template: Each component and its view is associated with an HTML template that declares how and what services the component offers. You may define the template inline or through file-path.
- Data binding: The different sections in a template interact with the components and their view through the process of data-binding. One must include binding markup in the HTML template so that Angular can connect with the component and template through the binding information.
- Dependency injection: DI helps in declaring the dependencies of the TypeScript classes to the new components while Angular takes care of the instantiation of those classes.
- Directives: Directives are classes in Angular that have the provision to add new behaviour in the elements of an application. There are three types of directives viz. components which are directives with a template; attribute directives that alter the appearance or functionality of an element, component, or another directive; and structural directives that add or delete DOM elements to change the HTML DOM layout.
- Metadata: Each class has metadata stored with the help of decorators. It helps in configuring how the class should process.
- Modules: A module refers to a block of code that divides your application into logical boundaries. Simply put, instead of coding all functionalities in a single application, you create separate Angular modules with distinct functionalities which together make the application. While it is ideal to have several modules, an Angular application must have at least one module, i.e. the root module.
- Routing: Routing in Angular provides the experience requested by the client. The user may choose an option on the main page that links to a URL. The router, which is bound to page links, renders the Angular component linked to that URL to the user.
- Services: You create a service in Angular when several modules require a common functionality or a value. For example, you would create a service for a database that is shared among various Angular modules.
4. Explain the following terms in Angular: expressions, scope and text interpolation.
- Angular expressions: Similar to Javascript, Angular expressions are blocks of code used to bind data to HTML. They are generally placed in binding i.e. inside curly braces like: {{ expression }}. Syntax: {{ expression }}
- Text interpolation: Interpolation means to embed expressions in HTML. Text interpolation enables you to include dynamic string values in the HTML templates, which means that you can dynamically change the text appearing on the application view. For example, greeting the users with their names on the welcome page. Text interpolation uses expressions within double curly {{ }} braces for displaying the component data.
- Scope: It is an object that Angular uses to bind controllers and views together. It defines the environment in which the expressions will be executed. The scope is arranged in a hierarchical structure similar to the application DOM structure.
5. What are some advantages and disadvantages of Angular?
Advantages of Angular framework:
- Supports two-way data-binding
- Exceptional community support
- Provision of adding custom directives
- Facilitation of client and server communication
- Supports dependency injection, validations and RESTful services
- Provides powerful features like event handling, animation, etc.
Disadvantages of Angular framework:
- Complex single-page applications are difficult and inefficient to use due to their bulkiness
- The dynamic application sometimes may not function as expected
- Becoming proficient in Angular requires a considerable amount of time and effort
6. What are the differences between Angular decorator and annotation?
Differences between Annotation and Decorator:
Annotation | Decorator |
Traceur compiler uses annotations | Typescript compiler uses the decorators |
The only metadata set on the class utilizing the Reflect Metadata library | Is a function that is called by the class |
Used to create an annotation array | Used to separate decoration or the modification in a class without changing any source code |
Angular annotations are hard-coded | Decorators are not Hard-coded |
Expression for imports for Annotations: import {ComponentAnnotation as Component} from ‘@angular/core’; | Expression for imports for Decorators: import {Component} from ‘@angular/core’; |
7. What is data binding and what are its types?
Data binding is one of the strong features of Angular that makes it easy for interactive applications to implement communication between HTML DOM and the Component Class. It automatically updates your page based on the latest application's state. Data binding is useful in specifying values like a source of an image, the state of a button, or any user-specific data.
You can customize your HTML by assigning attributes to the string values. For example in the below snippet ‘class’, ‘src’ and ‘disabled’ modify the ‘div’, ‘img’ and ‘button’ elements.
<div class="special">Data Binding Example</div> <img src="images/Hackertrail.png"></img> <button disabled>Save</button>
A point worth noticing here is that data binding does not work on the HTML attributes; instead, it can only be applied to the DOM element, component or directive properties. While DOM properties and HTML attributes have the same names, data binding distinguishes them as separate things. The DOM value represents the current value of a property while the HTML attribute is the property’s initial value.
There are three types of data binding in Angular depending on the direction of data flow:
- Interpolation binding- One way from data source to target view
- Event Binding- One way from view target to data source
- Two-way data binding
Intermediate Angular Interview Questions and Answers
8. Is it possible for an Angular application to render on the server-side?
Typical Angular applications are rendered on the browser i.e. on the client-side. However, to execute Angular applications on the server side, you can use a technology called Angular Universal.
Angular Universal generates static application pages which are bootstrapped on the browser when a client request comes in. Thus, it provides a great user experience by loading views faster and giving users instant access to the application view.
9. How can you communicate between application modules using core Angular functionalities?
The following core functionalities can be used to enable communication between Angular modules:
- Communication through events
- Via services
- By assigning models on $rootScope
- Directly between controllers [$parent, $$childHead, $$nextSibling, etc.]
- Directly between controllers [ControllerAs, or other forms of inheritance]
10. What is ahead-of-time(AOT) compilation in Angular? What are its advantages?
An Angular application primarily comprises components and their associated HTML templates. However, a browser can not decipher the components and templates by itself. Therefore, before running the Angular application on a browser, it needs to be compiled in a form understood by the browser. This is where the AOT or ahead-of-time compiler comes into play.
The Angular AOT compiler converts the HTML DOM and TypeScript code into browser readable JavaScript code during the build phase. The build phase takes place before the application is downloaded and executed by the browser.
Advantages of AOT compilation:
- Faster Rendering
- Fewer asynchronous requests
- Smaller Angular framework download size
- Detect template errors earlier
- Better security
11. What is MVVM in Angular?
The MVVM or Model View ViewModel is a refinement of the Model View Controller design pattern. In MVVM, the ViewModel facilitates the separation of the development of the Presentation Layer or the GUI (graphical user interface). This means that ViewModel (VM) converts the data objects in the model such that they are easier to manage and present. Thus, MVVM architecture makes the components loosely coupled.
Let us see each part of the MVVM design in detail:
- Model: It contains the structure of an entity, i.e., the data and the business logic of various modules of an application.
- View: This is a visual layer of the application that represents the data contained in the Model and hence consists of an HTML template of a component i.e. the UI code. It forwards the action initiated by the user to the ViewModel but does not get the response directly from ViewModel. Instead, it has to subscribe to the Observable exposed by that ViewModel.
- ViewModel: It is an abstract layer that becomes a bridge between the View and Model(business logic). It has no direct reference to the View and hence it cannot know which view is going to use it. Data-binding connects both View and ViewModel, therefore any change in either of them changes the data contained in the Model. To expose the Observable for View it has to interact with the Model.
12. What is Angular Bootstrap?
By default, an Angular application is initialised as it loads the angular.js script and places the ng-app directive automatically to your application’s root element. This is known as Automatic bootstrapping.
However, if there is a situation requiring you to initialize your application manually, you have to use the angular.bootstrap() function. For instance, you want the Angular application to initialize after a certain event has occurred and hence can use Angular bootstrap to achieve this. This is Manual bootstrapping which enables the developers to start the Angular application as per their requirement.
13. What is dirty-checking in Angular?
Dirty checking is a simple process of checking whether an unsynchronised value in the app has changed or not. In Angular, dirty checking scans the entire scope of a model or a component; i.e. the new scope values with the previous ones. All the scope variables are watched by Angular in a single loop, thus, any updates in one of the variables result in reassigning of remaining watched variables that exist in the DOM.
14. Explain the process of digest cycle in Angular
The digest cycle in Angular is when the watchlist is monitored to track changes in the watch variable value. Each digest cycle compares between the present version and previous versions of the scope model values.
The digest cycle process is implicitly triggered, however, one can start it manually too by using the $apply() function.
Advanced Angular Interview Questions and Answers
15. Explaining-app directive in Angular.
The ng-app directive tells Angular that this is the root element of an application and also enables developers to auto-bootstrap an application. It represents the root element of an Angular application and is generally declared near <html> or <body> tag. You can define multiple ng-app directives within an HTML document but implicitly only a single Angular application can be bootstrapped. The rest of the applications must be manually bootstrapped.
Here is an example of using ng-app:
<div ng-app=“myApp” ng-controller=“myCtrl”> Street1 : <input type=“text” ng-model=“streetAddr1”> <br /> Street2 : <input type=“text” ng-model=“streetAddr2”> <br> Full Address: {{streetAddr1 + ” ” + streetAddr1 }} </div>
16. How do Observable and Promise differ in execution?
Both Observable and Promise handle asynchronous tasks. However, there is a massive way in which both are executed.
- Observable is lazy in execution, i.e. they execute only after a subscription is made. Promise on the other hand is executed as soon as they are created.
- Observable is a stream of data that permits the passing of multiple events via a callback from a publisher to a subscriber. Whereas Promise handles only a single event.
- Observable is preferred over Promise as it has multiple operators. The developers can perform various operations on them like combining two Observable, piping multiple operations through an Observable, mapping one Observable into another one.
Cookies are small data packets that a browser uses to temporarily store user-related information. To be able to use cookies in Angular you need to use the following code:
npm install --save ngx-cookie-service
Inside the app.module.ts file, you need to include the CookieService class.
This CookieService class consists of several methods. Let us discuss the ones that are used to set, get, and clear cookies.
- The set() function is used to save or store information in a cookie. It takes two parameters, the cookie name and cookie value.
cookie.set('nameOfCookie',"cookieValue");
- To fetch a single value from a cookie, you use a get() method. And to get all values, you use the getAll() method. It takes a single parameter.
cookie.get(‘nameOfCookie’);
- There is a delete() function to delete or clear cookies. To delete a single key from the cookie you use delete(); to delete all the keys from cookies you can use deleteAll() method. It also uses a single parameter.
cookie.delete(‘nameOfCookie’);
To hide an HTML element on a button you have to use the ng-hide directive along with a controller as mentioned below:
View
<div ng-controller="HideElementController"> <button ng-click="hide()">Click here to hide</button> <p ng-hide="isHide">Poof! The element is gone</p> </div>
Controller
controller: function() { this.isHide = false; this.hide = function(){ this.isHide = true; }; }
19. What is an Angular Material Table?
The Angular Material Table is a comprehensive component that displays data in tabular form.
You may give your Material Table UI design feel or a Material Design look and feel. Read here to know more about how Material Tables function.
With this, we come to an end to our list of Angular interview questions. We have touched upon the topics that the interviewers generally look for in the candidates. However, to slay your Angular interview you must also be familiar with the programming languages like JavaScript, TypeScript and HTML as Angular uses them to create applications.
Also, you may visit the official website to learn more about Angular like setting up a local environment, working with a prebuilt Angular app, etc.
Backend Technology Interview Questions
C Programming Language Interview Questions | PHP Interview Questions | .NET Core Interview Questions | NumPy Interview Questions | API Interview Questions | FastAPI Python Web Framework | Java Exception Handling Interview Questions | OOPs Interview Questions and Answers | Java Collections Interview Questions | System Design Interview Questions | Data Structure Concepts | Node.js Interview Questions | Django Interview Questions | React Interview Questions | Microservices Interview Questions | Key Backend Development Skills | Data Science Interview Questions | Python Interview Questions | Java Spring Framework Interview Questions | Spring Boot Interview Questions.
Frontend Technology Interview Questions
HTML Interview Questions | JavaScript Interview Questions | CSS Interview Questions
Database Interview Questions
SQL Interview Questions | PostgreSQL Interview Questions | MongoDB Interview Questions | MySQL Interview Questions | DBMS Interview Questions
Cloud Interview Questions
AWS Lambda Interview Questions | Azure Interview Questions | Cloud Computing Interview Questions | AWS Interview Questions
Quality Assurance Interview Questions
Moving from Manual Testing to Automated Testing | Selenium Interview Questions | Automation Testing Interview Questions
DevOps and Cyber Security Interview Questions
DevOps Interview Questions | How to Prevent Cyber Security Attacks | Guide to Ethical Hacking | Network Security Interview Questions
Design Product Interview Questions
Product Manager Interview Questions | UX Designer Interview Questions
Interview Preparation Tips
Strength and Weakness Interview Questions | I Accepted a Job Offer But Got Another Interview | Preparation Tips For the Virtual Technical Interview | 7 Tips to Improve Your GitHub Profile to Land a Job | Software Engineer Career Opportunities in Singapore | What can you expect during a whiteboard interview | How To Write A Resignation Letter | Recommendation Letter Templates and Tips.
Quick Links
Practice Skills | Best Tech Recruitment Agency in Singapore, India | Graduate Hiring | HackerTrail Litmus | Scout - Sourcing Top Tech Talent in ONE Minute | About HackerTrail | Careers | Job Openings.
I’m horrible at Angular! Anyone come across any good tutorials?