C Programming Language Interview Questions and Answers 2023

To make any programming language interview successful, you must strengthen your foundational programming knowledge. C language is that foundation, and you must enhance this foundation to ace any coding interview. Dennis Ritchie developed this language for designing operating systems.
C is a high-level structured programming language that helps you learn other programming languages. That's why it is still prevalent during the interview process.
In this article, we will focus on the top commonly asked C Programming Interview Questions into the below sections:
Basic C Programming Language Interview Questions
- What are the five key features of the C programming language?
- What are the top 5 applications of C programming language?
- What do you mean by reserved words in C programming language?
- What do you mean by library functions?
- List the name of the tokens
- What is static() function in C programming?
- What is the difference between operators = and ==?
- What are 3 main drawbacks of C language?
- What is the difference between rvalue and lvalue?
- How does const char*p differ from the char const* p
Intermediate C Programming Language Interview Questions
- What is the importance of function?
- Explain the function prototype with an example.
- What do you mean by error? Discuss types of errors() in C language.
- What do you mean by function pointer?
- What is a header file in the C programming language?
- How can you implement decision-making processes in C language?
- What are the 4 primary sections to define a function in C language?
- What are modifiers? List the C programming modifiers
- What is an array, and why does it play a major role in C programming?
- Is sizeof() a function or operator?
Advanced C Programming Language Interview Questions
- Write the code to design a sequence of numbers/operators in which every row increases the object by one. In simple words – Pyramid pattern.
- What is a preprocessor? How can you use it in writing programs?
- Is it possible to convert a string to a number and vice versa?
- Explain recursion, a popular topic in C programming.
- How can you execute a program without main() function?
- What is the difference between macros and functions?
- Write a code to swap two numbers without using a third number.
- Without using multiplication operators, how can you multiply an integer number by 2?
- What are the different file opening modes?
- What would be the output of the following code?
Data Types and Variables
- What are the basic data types in C?
- What is the range of value that an ‘int’ data type variable can hold?
- What is the enumerated data type in C?
- Explain the String functions available in the C language
- What is typecasting in C?
- What is the difference between local and global variables?
- What is the difference between an actual parameter and a formal parameter?
- How can we store a negative integer?
Arrays, Strings, Functions, and Operators
- What is the difference in data storage between an array and a pointer?
- What is the difference between call by value and call by reference?
- Which function is used to compare two strings?
- What is the difference between a String and Array?
- Can we convert a number into String and vice-versa?
- Explain the difference between strncat() and strcat() in C.
- What are the different operators in C programming language?
- What is the difference between usage of the increment operator as ‘++i’ and ‘i++’?
- What are bitwise operators in C language?
Memory Management and Pointers
- What is a memory leak in C?
- Explain the difference between calloc() and malloc() functions.
- Explain the advantages and disadvantages of pointers in C
- What is a dangling pointer in C language?
- What is the difference between static and dynamic memory allocation in the C language?
- What is the difference between NULL and VOID pointers?
Libraries - File handling
- What are the different file functions in C?
- What is the difference between Random Access File and Sequential File in C?
- Explain the different file opening modes in C
- Why do we use the rewind() function in C?
Basic C Programming Language Interview Questions
1. What are the five key features of the C programming language?
The 5 key features are as follows:
- Machine Independent: The best feature of C programming language that makes your codes portable. You can write code on one platform and run it on other operating systems. This portability saves time and coding efforts.
- Rich libraries: This language has a rich set of in-build libraries, including functions, modules, and operators. You can use them in any required place.
- Simple & fast: C language allows you to write codes with simple syntax and rules. It makes the coding and its execution quick.
- Memory management: C language permits you to use its memory management functions and save memories by allocating them dynamically. Data structures and functions like malloc(), calloc() etc., support this.
- Extensible: You can easily extend the C programs into big projects.
2. What are the top 5 applications of C programming language?
C language is a general-purpose language which is why it is used in many applications like gaming, database designing, servers, etc. Still, the top 5 applications are as follows:
- Designing and developing operating systems – Operating system is a program which manages other application programs in computers. Unix, Microsoft Windows, and some android applications are all outcomes of C language.
- Designing Graphical User Interface (GUI) to establish interaction between the machine and human. The best example is Adobe Photoshop editors.
- Building compiler – a compiler has the main job of compiling the high-level language and making it executable for the machines. C language helps to build compilers so you can use one program on different platforms.
- Browser development – Without browsers, you can't access the internet. Credit goes to C language, which helps to design different browsers like Mozilla Firefox!
- Designing embedded system – An embedded system is an integration of hardware and software for implementing a particular function. C language makes it easy to create embedded systems.
3. What do you mean by reserved words in C programming language?
In any programming language, reserved words are those words that have been pre-defined and designed for particular use cases. You can't change the meaning of any reserved word and use it as an identifier name like function name or variable name. C programming language has over 30 reserved keywords, visible in the following table. For example, "long" is the keyword to define the data type, so you can't use it in the codes.
auto | break | case | char | const | int | float |
do | continue | switch | long | double | default | return |
short | signed | sizeof() | static | struct | typedef | unsigned |
void | while | if | goto | for | else | enum |
4. What do you mean by library functions in C programming language?
Library functions or built-in functions are functions that are pre-defined by the programming language. You can use those functions for different purposes. C language is enriched with hundreds of library functions. The main() is also a built-in function responsible for the code program execution.
Check the following table, which shows some popular library functions of header files "stdio.h" and "math.h"
Library - “studio.h”
printf() | It prints the output on the screen. |
scanf() | It takes input from users. |
getchar() | It takes character input from the users. |
gets() | It reads the line. |
Library - “Math.h”
pow() | It calculates the power of a number. |
sin() | It calculates the sine of a number. |
cos() | It calculates the cosine of a number. |
exp() | It calculates the exponent of a number. |
sqrt() | It calculates the square root of a number. |
5. List the name of the tokens.
In C or any other programming language, tokens are nothing but individual program elements. Here is a list of standard tokens:
- Keywords
- Identifiers
- Constant
- Operators
- Strings
- Special Characters
6. What is static() function in C programming?
The word "static" defines "fix", and you can't change. In C programming, static functions are fixed to a single file in which it is defined. You can restrict the function scope using static function as in C programming, all functions are by default global.
- You have to use the keyword "static" to make any function static.
- You can't call a static function outside of the defined function.
Check this link to get multiple examples of static functions in C programming.
7. What is the difference between operators = and == in C programming language?
= operator | == operator |
The operator "=" is the assignment operator used for assigning values. | The operator "==" is a comparison operator used for comparing two values. |
You can assign any constant at the LHS of this operator. | You can use constant values at both sides of this operator. |
{ int x; x = 10; //assign 10 to x } |
{ int a = 1; b = 2; printf(“Use of == operator:”, a == b) } It would compare the value of a and b. Since both are different, then it would return zero value. |
8. What are 3 main drawbacks of C language?
Even after having many features, C language has 3 main drawbacks, which are as follows:
- Lack of Object Oriented Programming concepts (OOPs) concept – These concepts are the pillars of modern programming languages. Its unavailability in C stops you from using it to develop modern systems and applications.
- No exception handling – You can't check and catch the exceptions in your C program codes. Exceptions are one of the critical programming concepts for developing error-free codes.
- Runtime checking – You have first to compile the code and run it to understand whether the program is accurate or not. You can't check any small error during compile time which increases the developing and execution time of whole codes.
9. What is the difference between rvalue and lvalue in C programming language?
lvalue | rvalue |
It is an object identifier that defines memory location to identify the object. | It is an expression that defines data value which would be stored at some memory address. |
It could be defined as either the LHS or RHS of the (=) operator. When it is on the LHS, it holds data value. Like int a; a = 1; But it is on the RHS, it meant modifiable l-values like expression. int a, b; a = 10; // int object & expression b = a; |
It should be defined at the RHS of the (=) operator. Like int d; d = 2; //d has r-value 2. |
10. How does const char*p differ from the char const* p
No, there is no difference between (const char* p) and (char const* p). Both refer to a constant pointer to a constant character. It means you can't change the values in either case.
Take this quiz to see how much you know about C programming!
Intermediate C Programming Language Interview Questions
11. What is the importance of function in C programming language?
Function refers to a group of statements to perform a specific task. It is useful in any programming language, especially in C programming because it not only provides large sets of built-in functions but also user-defined functions for executing many tasks. Here are other reasons why it is important:
- It allows you to remove big programs' complexity by breaking them down into small functions.
- It enhances the readability of programs so you can easily understand the codes.
- The top-down execution in C lets you learn the program flow and control every line.
- You can also create your header file and reuse it in other programs.
- You can test individual functions and make codes prune to fewer errors.
12. Explain the function prototype with an example.
Function prototype of prototype function refers to the declaration of any function with the following information:
- Name of function
- Function return type
- Number of arguments and their data type
The function prototype provides the assurance that the function call matches the return type and returns the correct number of arguments of the called function. Simply put, it helps prevent the user from making mistakes while using multiple functions in the program.
Syntax:
return_type function_name( datatype argument1, datatype argument 2, …);
Example
int sum(int x, int y);
This line is declaring the function "sum" which is taking two integer arguments. The function will also return the integer.
13. What do you mean by error? Discuss types of errors() in C language.
Errors are bugs that interrupt the program executions or don't generate accurate outcomes. You can consider them unexpected issues during code design and implementation. You can face 5 types of errors in C languages.
They are as follows:
- Syntax error: A design time error happens when you write codes and forget to follow pre-defined rules. The simplest example of this error is "forgetting to place a semicolon at the end of the statement." Compiler is responsible for finding this error.
- Logical error: An error that might happen because of incorrect logic applied in the code. This error present in the code can't generate the desired outcome. You have to search and find the flaw in the code as per requirements.
- Runtime error: An error that occurs during execution. You can quickly comply but are unable to execute because of incorrect logic, memory leak, incorrect input, or undefined variables.
- Semantic error: An error that happens because of incorrect implementation of codes. The best example is the type compatibility issue in the code. The compiler does not find any meaning in the code and interrupts the execution.
- Linker error: An error that happens during runtime because of errors in the executable files. Linker finds this error after the successful compilation of the code.
14. What do you mean by function pointer in C programming language?
In C programming language, a function pointer refers to a variable storing a function's memory addresses. The main aim of this concept is to store the function's memory address and call the function in the program without writing long code of the function again and again.
You have to use the asterisk symbol (*) to declare the function pointer and function parameters along with return type, and function arguments like this:
return type *function pointer (datatype argument 1, datatype argument 2, …)
Let's understand it with this dummy code:
int demo(int, int); //function int (*demp_ptr) (int, int); demo_ptr = demo // assigning the function address to function pointer //you can call the function as per requirement |
You can use function pointers in 2 ways like:
- Callback functions
- Functions as arguments to other functions
In any of these cases, you can create flexible functions and select the appropriate function behaviours.
Click this link to get different example codes for function pointers!
15. What is a header file in the C programming language?
The header file is one of the essential features in the C language. It refers to a file or place which consists of multiple functions to perform many tasks. The header file has the extension ".h" and contains 3 elements – function declaration, data type definitions, and macros.
You can't run any code without including header files. You have to use the preprocessor directive "#include" for this. Like this,
#include
It directs the compiler to use necessary header files before compilation. For example, #include
The studio.h is a common header file in every C program code to perform input/output operations. It consists of built-in functions including printf(), scanf(), getch(), etc.
Standard library and user-defined are two types of header files available in C programming.
16. How can you implement decision-making processes in C language?
The decision-making process in C programming language enables flow direction based on the conditions and assists in making decisions. Users need to decide according to certain conditions. For example, the government can give a driving licence if a person is 18+. To solve such problems, you need to use decision-making processes.
You can use the following options to implement it:
Options | Feature |
If statement | Helps you to make a simple decision-making process |
If-else statement | You can use it to check conditions and execute the required codes with alternative options. |
Nested-if statement | Sometimes, you need to check conditions within conditions. And in that case, use this option. |
If-else-if ladder | Use it if you have to check multiple conditions and get an alternative last option if none of them gets true. |
Switch | This is also an option to implement decisions by providing all essential options. |
Check the following image to learn every option through flow charts!
Image credit: Nerdyelectronics
17. What are the 4 primary sections to define a function in C language?
In C or any other programming language, you declare the required function in "function declaration" and define the function in "function definition".
In other words, you have to provide every detail of the function in the "function definition".
The following table shows its major sections.
Section name | Use |
Function Header | It should be identical to the function declaration except for the semicolon. You must define the function name, data type, arguments, and return type. |
Function body | It defines the set of executable statements whenever the function is called. |
Function name | Use the same function name as declared in the function header. |
Function Return type | What would be the return value of the function |
Check the following example to understand the function definition and its sections.
Int sum(int x, int y); // Function Declaration
int sum(int a, int b) //Function Header; //function name – sum // Return type - int
{
int c;
c = a + b;
printf(“Sum = %d”, c);
}
Whenever a function, sum() is called, users need to pass two integer numbers and it would return the sum of those number.
18. What are modifiers? List the C programming modifiers.
In C programming language, modifiers are keywords that change data types' meaning to modify the allocated memory for variables.
Modifiers have the following two main categories:
Size modifiers: These modify the size of the data type. It has subcategories: short and long
Sign modifiers: It changes the type of data type. It has subcategories: signed and unsigned
Modifier | Usage |
short | Use it when you to store small values of data type – int |
long | Use it when you have to increase the size of int/ double data types. |
signed |
Default modifier of datatypes: int & char You can use it for storing positive or negative value. |
unsigned | Supporting datatype is int and char but you can use it only for positive values. |
Check the following table to know every detail of these modifiers:
Data type | Storage (bytes) | Format specifier |
short int | 2 | %hd |
unsigned short int | 2 | %hu |
unsigned int | 4 | %u |
int | 4 | %u |
long int | 4 | %ld |
unsigned long int | 4 | %lu |
long long int | 8 | %lld |
unsigned long long int | 8 | %llu |
char | 1 | %c |
unsigned char | 1 | %c |
float | 4 | %f |
double | 8 | %lf |
Long double | 12 | %Lf |
19. What is an array, and why does it play a major role in C programming?
Array refers to a collection of values which possess a similar data type. For example, if "age" is an array, only age values (integer) will be stored in this array. An array doesn't permit the storage of different types of values. An array has the following special characteristics:
- Store data in contiguous memory locations
- Indexing starts from zero
- You can access array elements using their position
In C programming, mention array size while using array declaration to avoid errors. You get the following benefits when you use arrays in your C programs:
- You can store similar values in one place and use a variable to represent them.
- You can easily access to an array of elements through their location without considering their position
- You don't need to think about memory allocation because of continuous memory storage
- You can utilise other data structures like stack, queue, tree, graph, etc., with the help of an array
- You can create multiple arrays per requirements and apply functions to optimise codes.
20. Is sizeof() a function or operator?
In C programming, sizeof() is a unary operator, not a function. However, it looks like a function but plays the operator's role, which has the objective of computing the size of operands. You can use it with any data type.
Use cases | How it works | Examples |
Operand as data type() | When you use it with data types, it returns the allocated memory to that data types. | sizeof(int) = 4 sizeof(char) = 1 |
Operand as an expression | When you use it as an expression, it returns the size of the expression. |
int a = 1; float b = 1.0; sizeof(a+b) = 4 (as sum of a and b is 2.0 which is float so it returns 4) |
Besides, you can also use the sizeof()
- To calculate the number of elements in the array/list
- To allocate memory dynamically
C programming is the foundation. You should also be aware of the trends. Find out what the latest frontend development trends are by clicking this link.
Advanced C Programming Language Interview Questions
21. Write the code to design a sequence of numbers/operators in which every row increases the object by one. In simple words – Pyramid pattern.
#include <stdio.h>
int main() {
int i, j, rows;
printf("Enter the number of rows: ");
scanf("%d", &rows);
printf("Pyramid Design\n");
for (i = 1; i <= rows; ++i) {
for (j = 1; j <= i; ++j) {
printf("* ");
}
printf("\n");
}
return 0;
}
Output:
Enter the number of rows: 5
Pyramid Design
*
* *
* * *
* * * *
* * * * *
22. What is a preprocessor? How can you use it in writing programs?
In C programming language, preprocessor refers to the macro processor to define the macros (a small chunk of code or a kind of brief abbreviations). The C compiler calls it automatically to transform the program before compilation. You can use the preprocessor in the following ways:
- To include the header files essential to compile and run the codes.
- To define and expand the macros throughout the programs efficiently.
- To use it as line control which tells the compiler the origin of source lines.
- To use it for a conditional compilation that would help you to include or exclude specific parts of programs as per conditions.
Some examples: #define, #include, #if
23. Is it possible to convert a string to a number and vice versa?
Yes, it is possible to convert a string to a number and vice versa. The C language provides a built-in function, atoi(), that converts the string to an integer. To use this function, you must include the header file, stdlib.h.
Syntax:
int atoi (const char *string)
// you have to pass the string to the function, which will return the integer on successful execution. But, it returns 0 if the string is alphanumeric characters or starts with an alphanumeric character.
//In another case, if the string starts with a numeric character followed by an alphanumeric, the string is converted to an integer until the occurrence of the first alphanumeric character.
24. Explain recursion, a popular topic in C programming.
Recursion is a concept which is often used in a programming language. It allows you to call a function itself continuously. You have to put conditions to terminate the recursive function calls; otherwise, it will go to a loop.
In the following dummy code, you can see that a function, fact() is a recursive calling itself. And the main() function has declared it.
void fact() // recursive function which is calling itself
{
fact();
.
.
}
void main() // main function to define and declare the recursive function
{
.
.
fact ();
.
.
}
It is good to use this concept to write the codes for the task, which could be divided into sub-tasks. For example, the tasks like sorting, searching and traversing where you have to visit the list to find the specific number or location.
Tower of Hanoi, factorial, and Fibonacci are some evergreen problems where you can use recursion.
25. How can you execute a program without main() function?
Yes, executing a program without main() function in C is possible. You can use the preprocessor directive, #define, with arguments for this. This directive does not need any source code and you can run the program without the primary function.
Please refer to the following dummy code to understand it is done:
#include<stdio.h>
#define demo main
int demo(void)
{
printf(“you can execute a program without main() function”);
return 0;
}
Here, #define preprocessor directive is defining macro, which is replaced by the value of the macro.
26. What is the difference between macros and functions C programming language?
Macro | Function |
Macro is a small chunk of code which gets replaced whenever respective macros have been called. | A function is a group of one or more statements to perform a specific job. |
It is called a preprocessor directive, and it requires pre-processing. | It could be predefined or user-defined, and it needs compilation. |
It does not require any type-checking. | It requires type-checking. |
The increased number of macros increases the size of the whole program. | The function length does not impact the size of the program. |
Macro execution is faster than function execution. | The function execution is slower than the macro execution. |
Use macro when you need to execute small codes multiple times. | Use functions when executing large codes and different tasks in a program. |
27. Write a code to swap two numbers without using a third number.
#include <stdio.h>
int main()
{
int a=162, b=243;
printf("Before swap a=%d b=%d",a,b);
a=a+b;
b=a-b;
a=a-b;
printf("\nAfter swap a=%d b=%d",a,b);
return 0;
}
Output:
Before swap a=162 b=243
After swap a=243 b=162
28. Without using multiplication operators, how can you multiply an integer number by 2?
You can multiply or divide any integer number by 2 using bitwise operators. To perform multiplication without using * operator, you must use the left shift operator (<<) that shifts the bits left by 1.
Check the following code to understand how it works
#include <stdio.h>
int main()
{
int a;
printf("Enter any value for a:");
scanf("%d",&a);
printf("Value of a=%d",a);
printf("\nAfter multiplication by 2 using bitwise operator a=%d ",a<<1);
return 0;
}
Output:
Enter any value for a:25
Value of a=25
After multiplication by 2 using bitwise operator a=50
29. What are the different file opening modes in C programming language?
C language allows to open files in the following modes:
Mode | Functionality |
r | open a file in read mode |
w | open or create a text file in write mode |
a | open a file in append mode |
r+ | open a file in both read and write mode |
w+ | open a file in both read and write mode |
a+ | open a file in both read and append mode |
30. What would be the output of the following C language code?
#include <stdlib.h>
#include <stdio.h>
enum {false, true};
int main()
{
int i = 11;
do
{
printf("Value of i = %d\n", i);
i++;
if (i < 5)
continue;
}
while (false);
getchar();
return 0;
}
Output:
Value of i = 11
The above code will print the value of variable ‘I’. Since here do-while loop has been used, which executes at every iteration. While the loop will execute after the execution of the continue statement, since here the condition is false, thus it will print the value of ‘I’ only once.
Prepare for your virtual technical interview by clicking here.
Data Types and Variables C Programming Interview Questions
31. What are the basic data types in C programming language?
The basic data types in C are:
- Integer(int)
- Floating Point(float)
- Character(char)
- Void
Integer(int)
It is used to store whole numbers, may have below types depending on their sign and size:
- int/signed int
- unsigned int
- short int/ signed short int
- unsigned short int
- long int/ signed long int
- Unsigned long int
Floating Point(float)
They are used to store real numbers(with decimal points). Floating point data types in C have below types based on size:
- float
- double
- long double
Character(char)
They are used to store character values and can have below types based on sign:
- char/signed char
- unsigned char
Void
The void data type is used to depict “No value” when a function returns nothing to the calling function, it is specified using Void.
32. What is the range of value that an ‘int’ data type variable can hold?
Processor | Size of int | Range |
16-Bit | 2 Bytes | -32,768 to 32,767 |
32-Bit | 4 Bytes | -2,147,483,648 to 2,147,483,647 |
33. What is the enumerated data type in C programming language?
The enumerated data type is specified using keyword-’enum’. It is used for creating user-defined data types which have integral constants as their elements.
In the below example we have created an enum data type ‘week’ which consists of integral constants - Sunday, Monday, Tuesday, etc.
In the main program, we have created a variable called ‘today’ of enum data type ‘week’. This ‘today’ variable may store any one of the integral constants from the enum data type ’week’.
34. Explain the String functions available in the C language
C provides many string functions in its standard library header file- ‘string.h’. The most commonly used string functions are:
String Function | Description |
strlen(string_source) | Returns length of the string_source |
strcpy(string_destination, string_source) | Copies string_source into string_destination. |
strcat(string_destination, string_source) | Joins both the strings and stores the output in string_destination. |
strcmp(string_destination, string_source) | Compares both strings, if found equal returns 0(zero). |
strrev(string_source) | Reverses the string |
strlwr(string_source) | Returns the string into lowercase |
strupr(string_source) | Returns the string into uppercase |
35. What is typecasting in C programming language?
Typecasting refers to converting a variable’s data type into another data type explicitly by the programmer.
Type conversion can be performed in two ways:
Implicit type conversion:
Performed by the C compiler automatically, without any manual/programmer indication.
Example: It is performed by the compiler during the execution of an expression where two or more data types are present. The small data types are converted into large data types so that data is preserved without any loss.
Explicit type conversion or typecasting
It is deliberately performed by the programmer in the code by mentioning the required data type and this is known as typecasting in C.
Example: In the below example, we have deliberately changed the type of ‘c’ from float to int by writing the expected data type in brackets before the variable name ’c’.
36. What is the difference between local and global variables in C language?
Local Variable | Global Variable |
They are declared inside a function, their scope is limited to that particular function. | They are declared outside a function, their scope is throughout the program. |
When uninitialised, they store any garbage value. | When uninitialised, they store the default value as zero. |
To pass the value of local variables from one function to another, parameter passing is required. | Parameter passing is not required, because the scope is throughout the program, in all functions. |
If any changes are made to a local variable the changes are not reflected in other functions. | If any changes are made to a global variable the changes are reflected in all functions, at all places in the program. |
37. What is the difference between an actual parameter and a formal parameter in C language?
Formal Parameters | Actual Parameters |
These parameters are a list of variables. Here we define the data type of parameters which will be accepted by that function. | It is not a definition of parameters, here we provide the actual variables(parameters). |
They are mentioned in the function definition. | They are mentioned during the actual function call. |
The data type of the formal parameters is mentioned in the function definition. | Data types are not mentioned during making a call through actual parameters. |
Example:
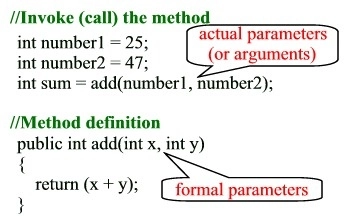
38. How can we store a negative integer in C language?
To derive a negative integer we calculate two’s complement of the positive number.
This can be done in a two-step process.
- Calculate one’s complement of the number.
- Add one to the complement to receive the negative number.
Example:
- Number 6 in binary is 0110
- Number 6’s one’s complement is 1001
- Add one to this 1001+1 = 1010 which is -6.
Arrays, Strings, Functions, and Operators C Programming Interview Questions
39. What is the difference in data storage between an array and a pointer in C language?
Below are the differences between an array and a pointer in storage:
Array | Pointer |
It is a collection of elements stored in memory continuously. | It stores the address of a variable. |
Memory allocation is sequential. | Memory allocation is dynamic. |
The memory allocation is static, once allocated cannot be changed in size or released. | The memory allocation is dynamic, the allocated memory can be resized or released. |
Memory is allocated at compile time. | Memory is allocated at runtime. |
40. What is the difference between call by value and call by reference in C language?
Call by value | Call by reference |
During function call when we pass variables by copying their values it is known as call by value. | During function call when we pass the address of the variables it is known as call by reference. |
If any changes are made to the values they are not reflected in the actual variables. | If any changes are made they are reflected in the actual variables because addresses were passed. |
41. Which function is used to compare two strings?
strcmp() can be used to compare two strings character by character and return an integer. It starts comparing the two strings character by character until the end of the string is reached which is null(‘\0’) or there is a mismatch.
42. What is the difference between a String and Array in C language?
Below are the differences between a String and Array in C:
String | Array |
It is a sequence of characters, whose end is denoted by NULL characters ('\0'). | It is a data structure, whose elements are of the same data type and accessed via index. |
String size can be changed if it is a char pointer. | The size of the array cannot be changed later. |
It can contain characters only. | It can contain characters, integers, floats, etc. |
43. Can we convert a number into String and vice-versa?
- We can use itoa() function to convert an integer data type into a string data type.
Prototype: char * itoa ( int value, char * str, int base ); Where : value is the integer to be converted to a string str is the resulting char array base is the numerical base of the integer value Returns a pointer to the char string.
- Similarly, we can use atoi() function to convert a string data type into an integer data type.
Prototype: int atoi (const char * str); Where str is a string constant which is received Returns the integer if the string is successfully converted, otherwise returns zero.
44. Explain the difference between strncat() and strcat() in C language.
strcat() | strncat() |
Syntax: strcat(source, destination) | Syntax: strncat(source, destination, number_of_characters) |
Concatenates destination string at the end of the source string. | Concatenates destination string at the end of the source string up to the number_of_characters mentioned. |
Find code snippets describing the differences between strncat() and strcat() below:
strcat(source, destination);
Example: #include<stdio.h> #include<string.h> #include<ctype.h> int main() { char src[]="Bombay"; char city[]="+Nagpur"; printf("Before concatenation destiona string = %s\n", src ); strcat(src, city); printf("After concatenation destination string = %s", src ); }
45. What are the different operators in C programming language?
There are 6 types of operators in C language:
1. Arithmetic
To perform arithmetic operations we use arithmetic operators.
Example:
2. Relational
To perform relational operations between operands.
Example:
3. Logical
To perform logical operations between operands.
Example:
4. Bitwise
To perform bit-by-bit operations on bits.
Example:
5. Assignment
To assign value to a variable.
Example:
6. Special
These are special or miscellaneous operators.
Example:
Read in detail about the operators present in the C language here.
46. What is the difference between usage of the increment operator as ‘++i’ and ‘i++’ in C language?
If we are using the operator ++ only with the operand and without assigning the value anywhere then in such cases, there is no difference in the usage and value of the operand.
Example 1
i=5; i++; printf(“%d”, i); Output is : 6
Example 2
i=5; ++i; printf(“%d”, i); Output again is: 6
The difference arises when we assign the operand into another variable after using the increment operator. This happens because operator precedence works on the expression and provides different output.
Example 3
i=5; j=i++; printf(“%d”, i);
Now, in this case, due to the fact that ‘=’ has higher precedence than postfix ‘++’, first the assignment is executed. So j is assigned the value of i, i.e. 5. After this assignment ‘++’ is executed, so the value of i is now 6.
i=6 and j=5.
Example 4
i=5; j=++i; printf(“%d”, i);
Now, in this case, due to the fact that the prefix ‘++’ has higher precedence than ‘=’, first the ‘++’ is executed. So i is incremented first and the value of i is 6. Now the next operation of assignment is executed, j is assigned the value of i , i.e. 6.
i=6 and j=6.
47. What are bitwise operators in C language?
Below are the bitwise operators in C with examples:
Let's assume A = 60, in binary : 0011 1100
B =13, in binary : 0000 1101
Operator | Description | Example |
& | Binary AND Operator copies a bit to the result if it exists in both operands. | (A & B) = 12, i.e., 0000 1100 |
| | Binary OR Operator copies a bit if it exists in either operand. | (A | B) = 61, i.e., 0011 0001 |
^ | Binary XOR Operator copies the bit if it is set in one operand but not both. | (A ^ B) = 49, i.e., 0011 0001 |
~ | Binary One's Complement Operator is unary and has the effect of 'flipping' bits. | (~A) = ~(60), i.e., 1100 0011 |
<< | Binary Left Shift Operator. The left operands value is moved left by the number of bits specified by the right operand. | A << 2 = 240, i.e., 1111 0000 |
>> | Binary Right Shift Operator. The left operands value is moved right by the number of bits specified by the right operand. | A >> 2 = 15, i.e., 0000 1111 |
Memory Management and Pointers C Programming Interview Questions
48. What is a memory leak in C language?
While writing an application, the developer has allocated some memory which he missed to deallocate after use. This results in unused allocated memory - and such phenomenon is referred to as a memory leak in C. Unused allocated memory could affect the system's performance and cause the speed to be slower. As a best practise, it is important to release all allocated memory after usage
49. Explain the difference between calloc() and malloc() functions in C language
malloc() and calloc() are functions to allocate memory dynamically at runtime. Below are the differences between the two:
malloc() | calloc() |
It allocates a single block of memory dynamically. | Allocates multiple blocks of memory dynamically. |
Memory allocated contains garbage values initially. | Memory allocated contains default value zero. |
Syntax: ptr = (cast_type *) malloc (byte_size); | Syntax: ptr = (cast_type *) calloc (n, size); |
Faster | Slower |
50. Explain the advantages and disadvantages of pointers in C language
Advantages of pointers in C:
- Pointers provide direct access to memory locations.
- They provide an alternate and better way to handle arrays.
- Pointers provide us the feature to dynamically allocate and deallocate memory.
- They help us to create and use complex data structures like trees, graphs, linked lists, stack, queue, etc.
Disadvantages of pointers in C:
- They are a complex feature to understand.
- If an incorrect value is updated in memory using pointers, this causes memory corruption.
- If not properly deallocated, pointers may cause a memory leak.
- They may cause a memory segmentation fault(accessing a non-existent memory location) if left uninitialised.’
You may read more about pointers here.
51. What is a dangling pointer in C language?
A dangling pointer in C is a normal pointer which is pointing to a memory location that is either deleted or deallocated.
In the below example the pointer ‘ptr’ is pointing to location ‘a’ which has address ‘5000’. Now later in the program, we released ‘a’ because it is a local variable of a function, but the ‘ptr’ is still pointing to ‘a’. Hence, ‘ptr’ is a dangling pointer now.
52. What is the difference between static and dynamic memory allocation in the C language?
Static memory allocation | Dynamic memory allocation |
Memory is allocated during compile time. | Memory is allocated during the runtime of the program. |
Once allocated we cannot change the memory size. | We can change the memory size according to need. |
Faster | Slower |
Memory remains allocated till the complete program runs. | We can release the memory in between the program run. |
Example: Array | Example: Tree |
53. What is the difference between NULL and VOID pointers in C language?
A null pointer is a pointer to any data type which is assigned a NULL value.
Void pointer is a data type itself, which remains void until some address reference is assigned to it. When we do not know the data type of the value which the pointer will point to, then we use a Void pointer.
Below are the differences between NULL and VOID pointers.
NULL | VOID |
A null pointer is a pointer that is not pointing to any data type currently. | A void is a pointer type that remains void until an address is assigned to it. |
It can be used to point data types int, float, char, etc. according to its own type. | It is a generic pointer which can point to any data type. |
Libraries - File handling C Programming Interview Questions
54. What are the different file functions in C language?
Function name | Description of the function |
fopen() | opens a new or an existing file |
fprintf() | write data into a file |
fscanf() | reads data from a file |
fputc() | writes a character into a file |
fgetc() | reads a character from a file |
fclose() | closes a file |
fseek() | sets the file pointer to a given position |
fputw() | writes an integer to a file |
fgetw() | reads an integer from a file |
ftell() | returns the current position of the file pointer |
rewind() | sets the file pointer to the beginning of a file |
55. What is the difference between Random Access File and Sequential File in C language?
When we want to access data from a sequential file, we need to traverse the data sequentially from the start till we reach the required data record.
However, in a random access file, we can access the data randomly, without traversing the whole file. Therefore, random access files are faster to access, read and modify data.
56. Explain the different file opening modes in C language
Below are the different file opening modes in C:
File Mode | Description |
r | Open a file for reading. If a file is in reading mode, then no data is deleted if a file is already present on a system. |
w | Open a file for writing. If a file is in writing mode, then a new file is created if a file doesn't exist at all. If a file is already present on a system, then all the data inside the file is truncated, and it is opened for writing purposes. |
a | Open a file in append mode. If a file is in append mode, then the file is opened. The content within the file doesn't change. |
r+ | Open for reading and writing from beginning |
w+ | Open for reading and writing, overwriting a file |
a+ | Open for reading and writing, appending to file |
57. Why do we use the rewind() function in C language?
Rewind() is used to set the file pointer to the beginning of the file.
Syntax:
void rewind(FILE *stream);
This function does not return any value. We should include the ‘stdio.h’ header file in the program to use this function.
Final Thoughts
It doesn’t matter whether a company is a big IT one or a small software firm, they all seek software professionals with C coding skills. With these questions, you can sharpen yourbasic programming knowledge. Join our platform to practise coding skills, take quizzes, and prepare for interviews. Share your questions in the comment section.
Other Backend Technology Interview Questions and Answers
PHP Interview Questions | .NET Core Interview Questions | NumPy Interview Questions | API Interview Questions | FastAPI Python Web Framework | Java Exception Handling Interview Questions | OOPs Interview Questions and Answers | Java Collections Interview Questions | System Design Interview Questions | Data Structure Concepts | Node.js Interview Questions | Django Interview Questions | React Interview Questions | Microservices Interview Questions | Key Backend Development Skills | Data Science Interview Questions | Python Interview Questions | Java Spring Framework Interview Questions | Spring Boot Interview Questions.